Introduce Aspida
Let's try sending HTTP requests from the client to the Fastify server we have set up on the previous page.
By the way, if you use common HTTP clients such as fetch or axios, the response type will be any
, and you will not be able to achieve 'One TypeScript'.
Therefore, we use aspida, a TypeScript-friendly HTTP client wrapper.
TypeScript friendly HTTP client wrapper for the browser and node.js.
Star1. Specify API Type Definition
1.1. Create API Definition Files
Back to frourio-tutorial
directory, and prepare api type definitions.
mkdir -p src/api/hi
yarn add aspida
Create and edit src/api/index.ts
and src/api/hi/index.ts
as follows.
import { DefineMethods } from 'aspida';
export type Methods = DefineMethods<{
get: {
resBody: {
hello: string;
};
};
}>;
We recommend to use DefineMethods
with semicolon in order to get proper editor support.
1.2. Prepare Aspida Config File
Create and edit aspida.config.js
as follows.
module.exports = {
input: 'src/api',
baseURL: 'http://localhost:8888',
};
1.3. Generate Object with Aspida
Next, generate api definition with aspida.
Aspida, a CLI tool, converts api definition files corresponding to each path into a single object.
yarn aspida
Aspida generates api/$api.ts
.
This file contains information about endpoints and type definitions of each HTTP method for them as the object hierarchy.
1.4. Read $api.ts
File (Optional)
If you already know about aspida, you can skip this section.
Let's take a look at api/$api.ts
.
In this file, there is a function named api
. This function returns an object with information about endpoints.
Since all this information is fully typed, we can call the endpoints type-safe.
2. Send API Requests
2.1. Create API Client
To send api requests, use an HTTP client wrapper such as @aspida/fetch
or @aspida/axios
.
In this tutorial, we use @aspida/fetch
.
yarn add @aspida/fetch
Next, create and edit src/apiClient.ts
as follows.
import api from './api/$api';
import aspida from '@aspida/fetch';
const apiClient = api(
aspida(undefined, {
baseURL: 'http://localhost:8888',
}),
);
export default apiClient;
2.2. Fetch from Server
Then, edit src/App.tsx
as follows.
-import React from 'react';
+import React, { useEffect, useState } from "react";
+import api from "./apiClient";
function App() {
+ const [greeting, setGreeting] = useState("loading...");
+
+ useEffect(() => {
+ api.hi.$get().then((res) => {
+ setGreeting(res.hello);
+ });
+ }, []);
Here we have api response in greeting
.
2.3. Display Greeting
All that remains is to display it.
<p>
Edit <code>src/App.tsx</code> and save to reload.
</p>
+ <p>{greeting}</p>
yarn start
How are you?
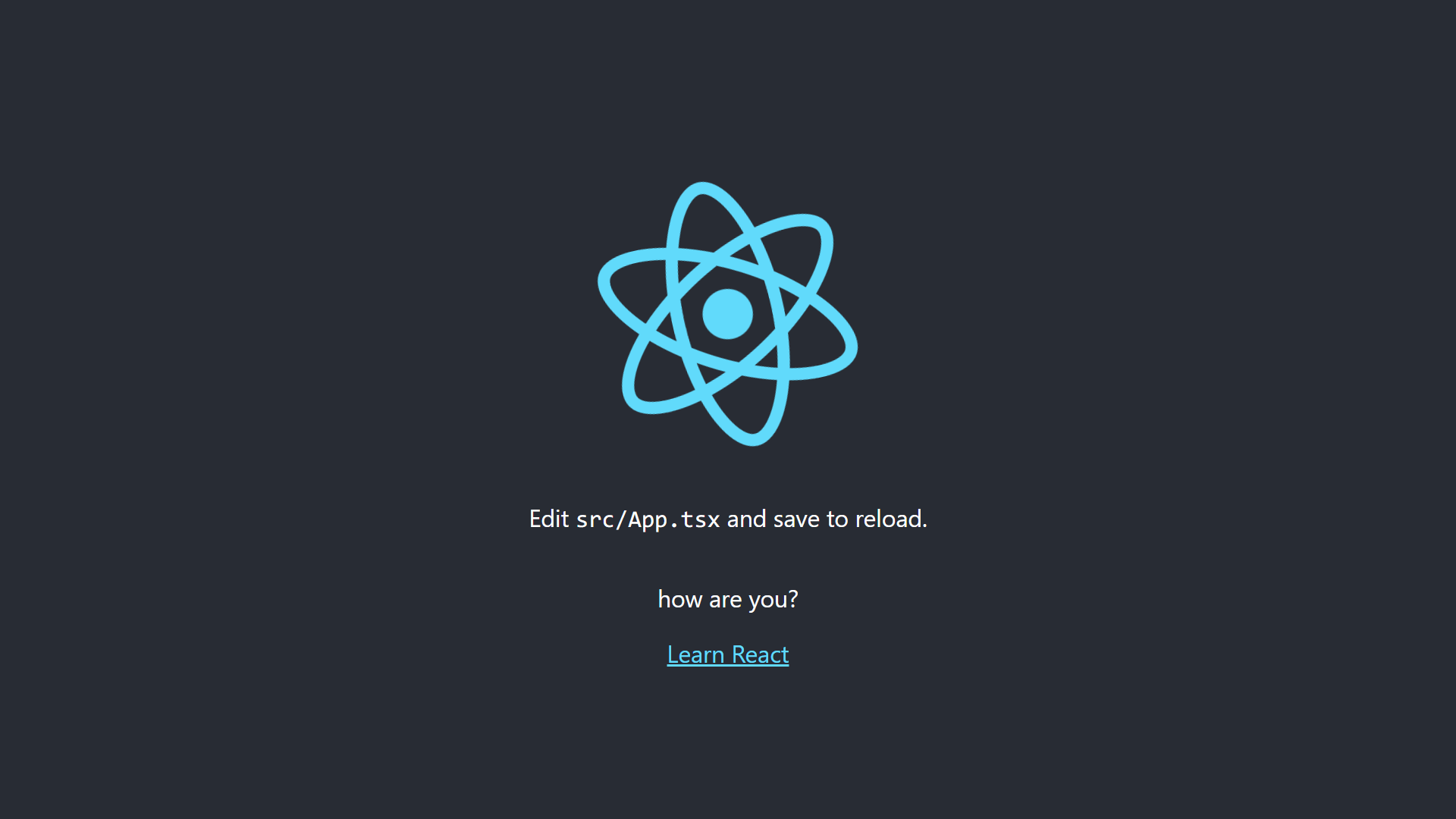
Check the following.
- Did you start the back-end server? (
yarn ts-node index.ts
infrourio-tutorial/server
directory) - Did you start the front-end server? (
yarn start
infrourio-tutorial
directory) - Did you generate the API file? (
yarn aspida
infrourio-tutorial
directory)